Notes app is the best place to jot down quick thoughts or to save longer notes filled with checklists, text or web links.
And this article is creating a Notes app using HTML, CSS, and JavaScript. And source code, you can get from (Tilak Jain): GitHub
HTML/CSS/JavaScript contents:
1. Create an HTML (index.html)
<!DOCTYPE html>
<html>
<body>
<div class="container my-3">
<h1>My Notes App</h1>
<div class="card">
<div class="card-body">
<h5 class="card-title">Add a note</h5>
<div class="form-group">
<textarea class="form-control" id="addTxt" rows="3"></textarea>
</div>
<button class="btn btn-primary" id="addBtn">Add Note</button>
</div>
</div>
<hr>
<h1>Your Notes</h1>
<hr>
<div id="notes" class="row container-fluid"> </div>
</div>
</body>
</html>
2. CSS StyleSheet, Add bootstrap link in HTML file
https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css
3. Create JavaScript (script.js)
showNotes();
// If user adds a note, save it to the localStorage
let addBtn = document.getElementById("addBtn");
addBtn.addEventListener("click", function(e) {
let addTxt = document.getElementById("addTxt");
let notes = localStorage.getItem("notes");
if (notes == null) {
notesObj = [];
} else {
notesObj = JSON.parse(notes);
}
notesObj.push(addTxt.value);
localStorage.setItem("notes", JSON.stringify(notesObj));
addTxt.value = "";
showNotes();
});
// Function to show elements from localStorage
function showNotes() {
let notes = localStorage.getItem("notes");
if (notes == null) {
notesObj = [];
} else {
notesObj = JSON.parse(notes);
}
let html = "";
notesObj.forEach(function(element, index) {
html += `
<div class="noteCard my-2 mx-2 card" style="width: 18rem;">
<div class="card-body">
<h5 class="card-title">Note ${index + 1}</h5>
<p class="card-text"> ${element}</p>
<button id="${index}"onclick="deleteNote(this.id)" class="btn btn-danger">Delete Note</button>
</div>
</div>`;
});
let notesElm = document.getElementById("notes");
if (notesObj.length != 0) {
notesElm.innerHTML = html;
} else {
notesElm.innerHTML = `Nothing to show! Create your first Note!`;
}
}
// Function to delete a note
function deleteNote(index) {
let notes = localStorage.getItem("notes");
if (notes == null) {
notesObj = [];
} else {
notesObj = JSON.parse(notes);
}
notesObj.splice(index, 1);
localStorage.setItem("notes", JSON.stringify(notesObj));
showNotes();
}
Complete code:
<!DOCTYPE html>
<html>
<body>
<!-- bootstrap cdn -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" integrity="sha384-ggOyR0iXCbMQv3Xipma34MD+dH/1fQ784/j6cY/iJTQUOhcWr7x9JvoRxT2MZw1T" crossorigin="anonymous">
<div class="container my-3">
<h1>My Notes App</h1>
<div class="card">
<div class="card-body">
<h5 class="card-title">Add a note</h5>
<div class="form-group">
<textarea class="form-control" id="addTxt" rows="3"></textarea>
</div>
<button class="btn btn-primary" id="addBtn">Add Note</button>
</div>
</div>
<hr>
<h1>Your Notes</h1>
<hr>
<div id="notes" class="row container-fluid"> </div>
</div>
<script src="./script.js"></script>
</body>
</html>
Final Result:
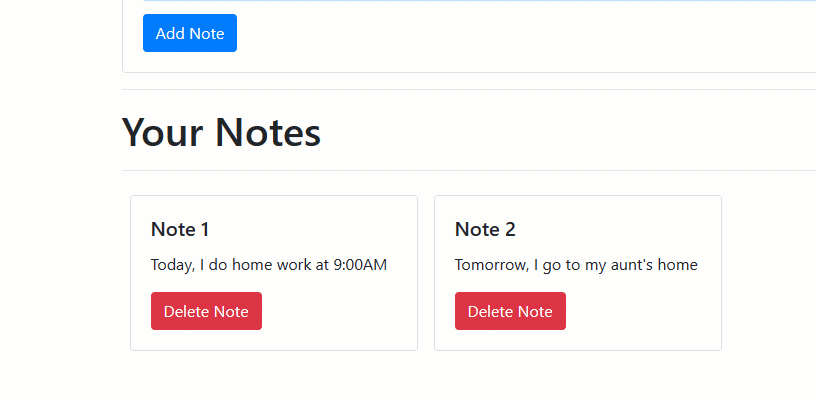
JavaScript – Password Generator
A password generator is a tool that automatically generates passwords based on policies you set, creating strong and unpredictable passwords for each of your accounts.
And this article is creating a Password Generator app using HTML, CSS, and JavaScript.
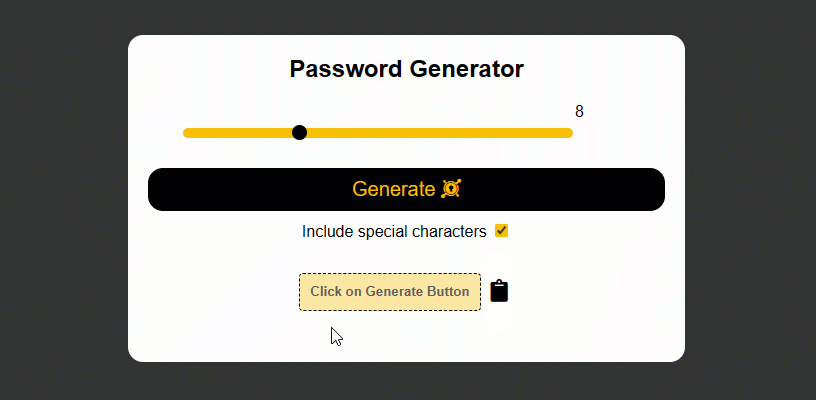
JavaScript – Counter App
You can create an app for counting numbers using JavaScript to help users count items or products or something.
And this article is creating a Counter app using HTML, CSS, and JavaScript.
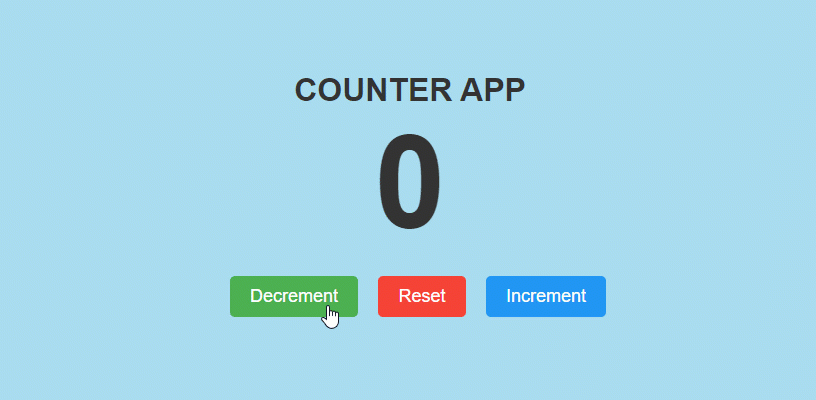
Related Articles
- Automatically Setting the Current Date and Time in an HTML Form
- How to Create a Spinner with Loading Data Percentage in DataTables
- Convert Numbers to Khmer Number System Words (HTML+JAVASCRIPT)
- Converting Numbers to Words in JavaScript
- Create Login Form Using HTML, CSS, JavaScript
- JavaScript – Create Notes App