A login form is a graphical user interface (GUI) element used to collect user authentication information, such as a username and password, to gain access to a restricted area or a security system.
This article shows you how to create a simple login form using HTML, CSS, and JavaScript. It’s just basic concept ideas to help you build a website or application.
HTML Tutorials Contents
Create a login form (login.html)
A typical login form consists of two input fields – one for the username and the other for the password – along with a “submit” button.
<!DOCTYPE html>
<html>
<head>
<title>Login Form</title>
</head>
<body>
<form>
<h2>Login</h2>
<label for="username">Username:</label>
<input type="text" id="username" name="username">
<br>
<label for="password">Password:</label>
<input type="password" id="password" name="password">
<br>
<button type="button" onclick="login()">Login</button>
</form>
</body>
</html>
Create a CSS file (style.css)
form {
border: 2px solid #ccc;
border-radius: 5px;
padding: 20px;
width: 300px;
margin: 0 auto;
}
label {
display: block;
font-weight: bold;
margin-bottom: 10px;
}
input[type=text],
input[type=password] {
padding: 5px;
width: 100%;
border-radius: 3px;
border: 1px solid #ccc;
margin-bottom: 20px;
}
button {
background-color: #4CAF50;
color: white;
padding: 10px;
border: none;
border-radius: 5px;
cursor: pointer;
width: 100%;
}
button:hover {
background-color: #45a049;
}
Create a Javascript (script.js)
JavaScript file creates an event on the Login button to check and verify username with password condition. So when you fill username and password ready, you click on the Login button it will alert the message “Invalid username or password” or “Login successful!” with username=”admin” and Password=”admin”.
function login() {
var username = document.getElementById("username").value;
var password = document.getElementById("password").value;
// Your login validation logic here
if (username == "admin" && password == "admin") {
alert("Login successful!");
} else {
alert("Invalid username or password.");
}
}
Login form link to CSS and JavaScript
To link an HTML page with a CSS file and JavaScript file, add:
StyleSheet link between header tag <head>
<link rel="stylesheet" type="text/css" href="style.css">
JavaScript link out of form tag
<script src="script.js"></script>
For complete code
<!DOCTYPE html>
<html>
<head>
<title>Login Form</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<form>
<h2>Login</h2>
<label for="username">Username:</label>
<input type="text" id="username" name="username">
<br>
<label for="password">Password:</label>
<input type="password" id="password" name="password">
<br>
<button type="button" onclick="login()">Login</button>
</form>
<script src="script.js"></script>
</body>
</html>
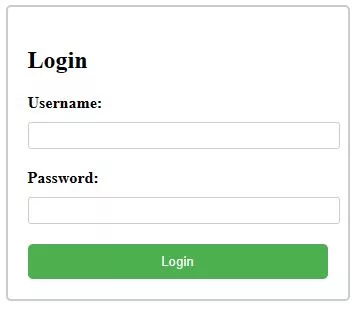
Related Articles
- Automatically Setting the Current Date and Time in an HTML Form
- How to Create a Spinner with Loading Data Percentage in DataTables
- Convert Numbers to Khmer Number System Words (HTML+JAVASCRIPT)
- Converting Numbers to Words in JavaScript
- Create Login Form Using HTML, CSS, JavaScript
- JavaScript – Create Notes App