With android studio, a dialog is a small window that prompts the user to make a decision or enter additional information. Developers can customize dialog messages with some interface style. It pop-up on the screen like a Simple Toast message but it’s not pop up shortly, it requires the user to press for closing. And A Toast message just informs you of information, but the dialog message requires you a decision.
For this article, I show you how to create a dialog message Yes/No message in the android studio, So please follow with me step by step :
1 – Create a new android project
For this example, the project name is mynewapp
2 – In the activity_main.xml
In the layout (activity_main.xml), add a button.
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/btn_exit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Exit"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
3 – In the MainActivity.java
package com.eangkor.mynewapp;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.content.ContextCompat;
import android.app.AlertDialog;
import android.content.Context;
import android.content.DialogInterface;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
Button btnExit;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btnExit=findViewById(R.id.btn_exit);
// On click event on button
btnExit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showSimpleDialog();
}
});
}
private void showSimpleDialog(){
AlertDialog.Builder builder;
builder = new AlertDialog.Builder(this);
builder.setMessage("Do you want to exit?");
//Setting message manually and performing action on button click
builder.setMessage("Do you want to exit?")
.setCancelable(false)
.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
// Action for 'Yes' Button
//exit application
finish();
System.exit(0);
}
})
.setNegativeButton("No", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
// Action for 'No' Button
Toast.makeText(getApplicationContext(),"Cancel",Toast.LENGTH_SHORT).show();
}
});
//Creating dialog box
AlertDialog alert = builder.create();
//Setting the title manually
alert.setTitle("Message Title");
alert.show();
}
}
Final Result :
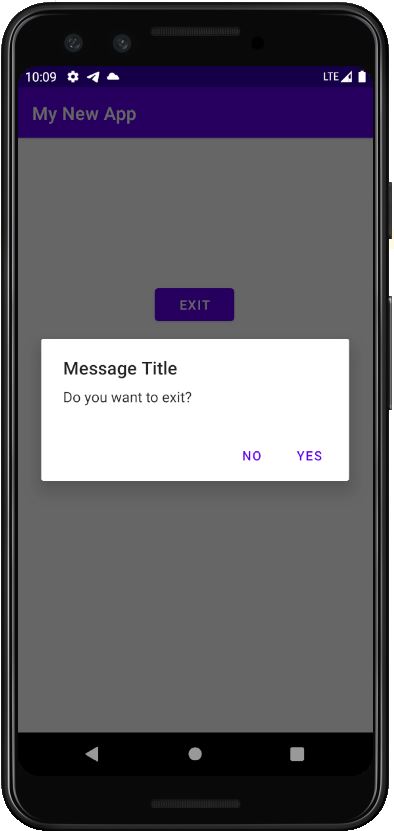
You need to take part in a contest for one of the highest quality sites on the net. I am going to recommend this site!