With android, the Toast message is important for informing the message user. It displays information with a small popup and short period, and you can customize this Toast message with some style, and in this article, I show you how to create a simple toast message and custom toast message. For a simple toast message, you can see it on the bottom of the screen side. And I have two methods to show you below.
Android Contents:
How to create simple a toast message in Android
So please follow with me step by step :
The first time, you create a new project in Android Studio, for example, project name : mynewapp
android.widget.Toast; class is the subclass of java.lang.Object class. So you should import android.widget.Toast;
import android.widget.Toast;
Simple toast message
Method 1
Toast.makeText(getApplicationContext(),"Hello android",Toast.LENGTH_SHORT).show();
Method 2
Context context = getApplicationContext();
CharSequence text = "Hello android";
int duration = Toast.LENGTH_SHORT;
Toast.makeText(context, text, duration).show();
For example: create a toast message at MainActivity.java when onCreate
So when you open the app, a Toast message will display at bottom of your device screen.
package com.eangkor.mynewapp;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.os.Bundle;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Context context = getApplicationContext();
CharSequence text = "Hello android";
int duration = Toast.LENGTH_SHORT;
Toast.makeText(context, text, duration).show();
}
}
Final Result :
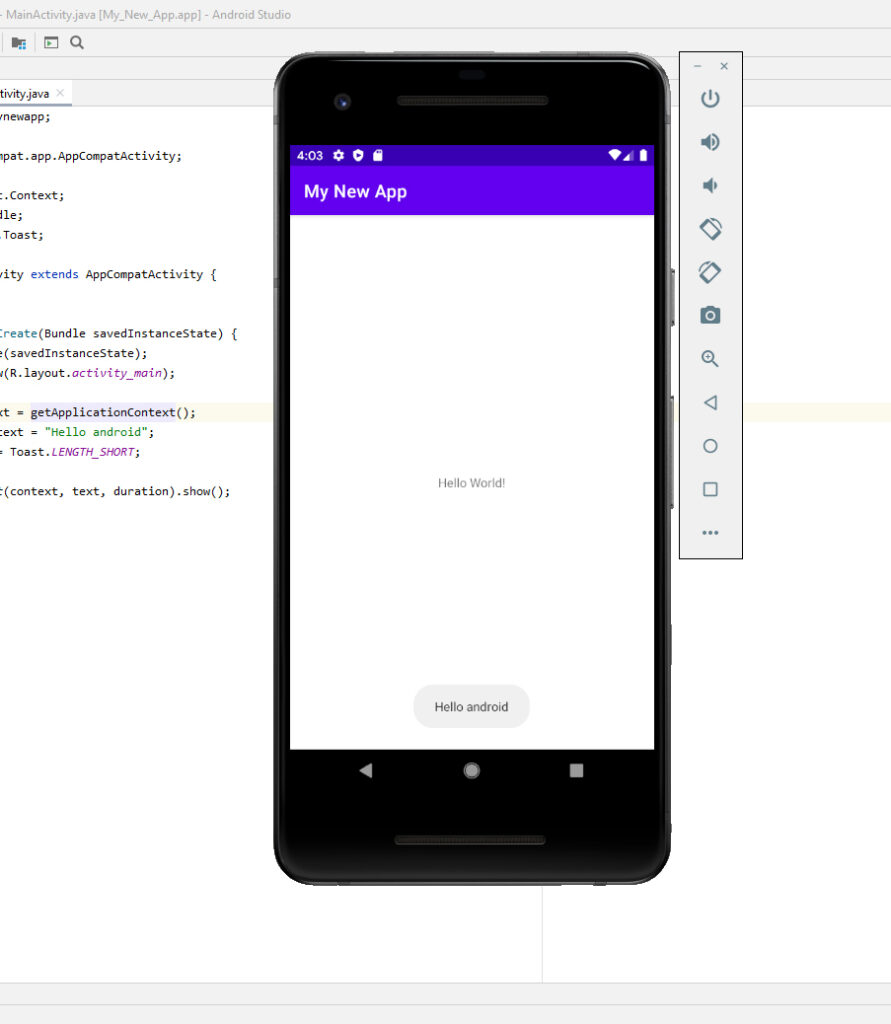
How to create a custom toast message in android
In Android, toast is important for informing the user about an information message.
1- Create a new Android project
Project name: Custom Toast Message
Package name: com.eangkor.customtoastmessage
Language: Java
2- activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/btn_showtoast"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Show Toast"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
3- customtoastmessage.xml
Right-click on the Layout and choose New and then select on Layout Resource File. At the File Name : customtoastmessage and click on OK
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/custom_toast_layout"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/custom_toast_image"
android:layout_width="63dp"
android:layout_height="44dp"
android:layout_marginBottom="16dp"
android:src="@drawable/ic_action_name"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toStartOf="@+id/custom_toast_message"
app:layout_constraintHorizontal_bias="0.919"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.988" />
<TextView
android:id="@+id/custom_toast_message"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="16dp"
android:contentDescription="To"
android:text="Welcome to app"
android:textColor="#009688"
android:textSize="30dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.666"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.988" />
</androidx.constraintlayout.widget.ConstraintLayout>
4- MainActivity.java
package com.eangkor.customtoastmessage;
import androidx.appcompat.app.AppCompatActivity;;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
Button btnshowtoast;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btnshowtoast=(Button)findViewById(R.id.btn_showtoast);
btnshowtoast.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
LayoutInflater li = getLayoutInflater();
View layout = li.inflate(R.layout.customtoastmessage,(ViewGroup) findViewById(R.id.custom_toast_layout));
Toast toast = new Toast(getApplicationContext());
toast.setDuration(Toast.LENGTH_SHORT);
toast.setView(layout);
toast.show();
}
});
}
}
Final Result:
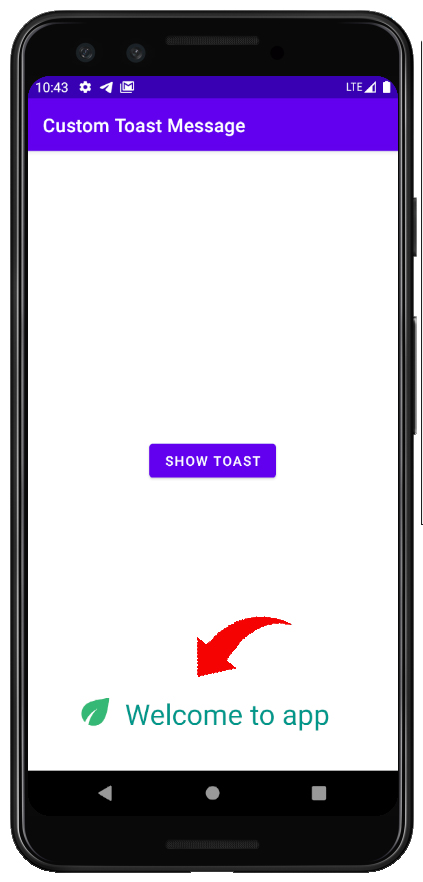
You need to take part in a contest for one of the highest quality sites on the net. I am going to recommend this site!