A placeholder is a temporary or initial piece of text or visual cue that is displayed in a field or input element, typically to provide instructions or indicate the type of information expected. It serves as a guide or prompt for users to enter the appropriate data in a form or input field.
Excel Contents:
But If you want to run your project, you must save your Excel project to Excel Macro – Enable Workbook (*.xlsm)
Set a placeholder on a TextBox in Excel VBA Without Form
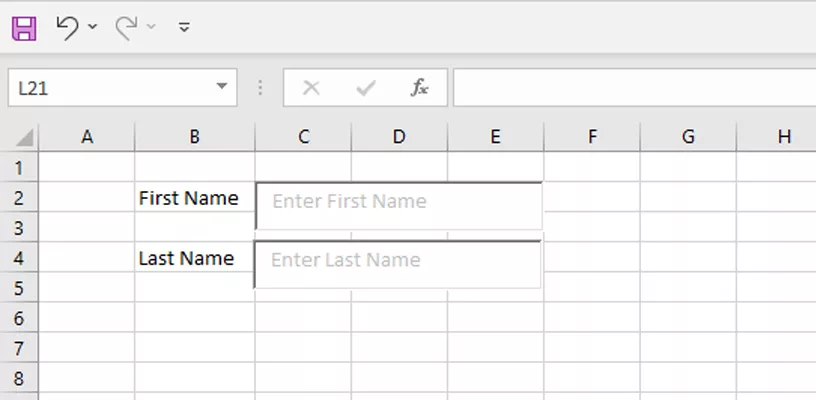
Private Sub TextBox1_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single)
If TextBox1.Value = "Enter First Name" Then
TextBox1.ForeColor = &H80000008
TextBox1.Value = ""
End If
End Sub
Private Sub TextBox2_MouseDown(ByVal Button As Integer, ByVal Shift As Integer, ByVal X As Single, ByVal Y As Single)
If TextBox2.Value = "Enter Last Name" Then
TextBox2.ForeColor = &H80000008
TextBox2.Value = ""
End If
End Sub
Private Sub TextBox1_LostFocus()
If TextBox1.Value = "" Then
TextBox1.Value = "Enter First Name"
TextBox1.ForeColor = &HC0C0C0
End If
End Sub
Private Sub TextBox2_LostFocus()
If TextBox2.Value = "" Then
TextBox2.Value = "Enter Last Name"
TextBox2.ForeColor = &HC0C0C0
End If
End Sub
Set a placeholder on a TextBox in Excel VBA Form
In the context of Excel VBA, a placeholder on a TextBox can be used on UserForm, So when you enter on a TextBox it will clear placeholder value to blank TextBox.
How you can create a placeholder effect for a TextBox in Excel VBA:
1. Insert a TextBox control on your worksheet. Right-click on the TextBox and select “Properties” from the context menu.
2. In the Properties Window, set the TextBox’s Value
property to the desired placeholder text. For example, “Enter your name here.”
3. Add the following VBA code to the worksheet module (e.g., right-click on the worksheet tab and choose “View Code”):
Private Sub TextBox1_Enter()
If TextBox1.Value = "Enter Your Name" Then
TextBox1.Value = ""
End If
End Sub
Private Sub TextBox1_Exit(ByVal Cancel As MSForms.ReturnBoolean)
If TextBox1.Value = "" Then
TextBox1.Value = "Enter Your Name"
End If
End Sub
In this example, when the TextBox receives focus (Enter event), the code checks if the TextBox value is equal to the placeholder text. If it matches, the code clears the TextBox to allow the user to enter their own text.
When the TextBox loses focus (Exit event), the code checks if the TextBox is empty. If it is, it sets the TextBox value back to the placeholder text.
Example Form With Code
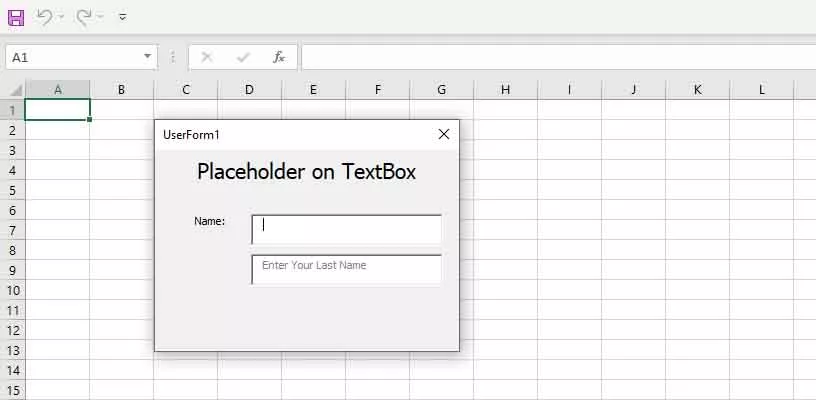
Private Sub UserForm_Initialize()
TextBox1.Value = "Enter Your First Name"
TextBox2.Value = "Enter Your Last Name"
If TextBox1.Value = "Enter Your First Name" Or TextBox2.Value = "Enter Your Last Name" Then
TextBox1.ForeColor = RGB(128, 128, 128)
TextBox2.ForeColor = RGB(128, 128, 128)
End If
End Sub
Private Sub TextBox1_Enter()
If TextBox1.Value = "Enter Your First Name" Then
TextBox1.Value = ""
TextBox1.ForeColor = RGB(0, 0, 0)
End If
End Sub
Private Sub TextBox1_Exit(ByVal Cancel As MSForms.ReturnBoolean)
If TextBox1.Value = "" Then
TextBox1.Value = "Enter Your First Name"
TextBox1.ForeColor = RGB(128, 128, 128)
End If
End Sub
Private Sub TextBox2_Enter()
If TextBox2.Value = "Enter Your Last Name" Then
TextBox2.Value = ""
TextBox2.ForeColor = RGB(0, 0, 0)
End If
End Sub
Private Sub TextBox2_Exit(ByVal Cancel As MSForms.ReturnBoolean)
If TextBox2.Value = "" Then
TextBox2.Value = "Enter Your Last Name"
TextBox2.ForeColor = RGB(128, 128, 128)
End If
End Sub
Some suggestion:
Please note that this approach is specific to an ActiveX TextBox control on a worksheet. If you are working with a UserForm in Excel VBA, you can use the ControlTipText
property as described in the previous response.